- Buffer overflow
- RAM injection
- RAM spoofing
- SQL injection
For more questions and Answers:
The correct answer is Buffer Overflow. Let me expand on this concept in detail.
Introduction Buffer Overflow
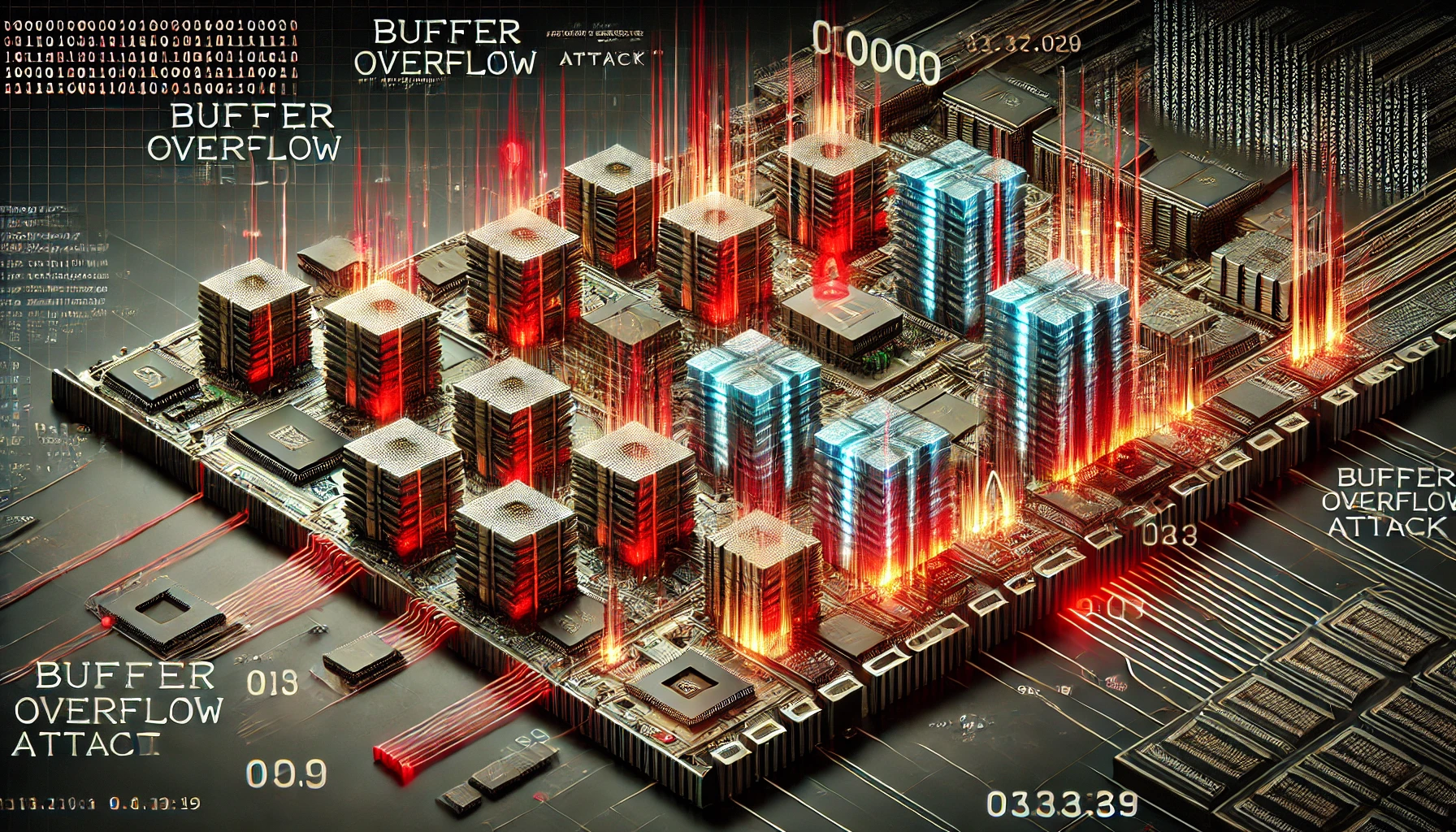
A buffer overflow is a type of cyber attack that occurs when data written to a buffer exceeds the allocated memory space. This vulnerability is common in applications written in programming languages like C and C++ that lack inherent bounds-checking mechanisms. Exploiting buffer overflows allows attackers to overwrite memory, corrupt data, or inject malicious code.
Buffer overflow vulnerabilities are among the oldest and most dangerous forms of security risks, classified under the CWE-120 (Common Weakness Enumeration) as “Buffer Copy without Checking Size of Input.”
To understand buffer overflows in-depth, we need to explore how memory works, the nature of buffers, and how attackers exploit this weakness.
What is a Buffer?
A buffer is a temporary storage area in memory used to hold data. Buffers are commonly used in programming to store strings, arrays, or other types of data during execution. For instance:
- Input Buffers store user input, such as strings.
- Output Buffers temporarily hold output data before it is sent.
When a program writes data to a buffer, it must ensure that the size of the data does not exceed the memory allocated for that buffer. Failure to validate this size leads to a buffer overflow.
How Buffer Overflow Occurs
A buffer overflow happens in the following scenario:
- A buffer (e.g., an array or string) is allocated a fixed size in memory.
- The application writes data to the buffer without checking its size.
- If the input data is larger than the buffer, the excess data overflows into adjacent memory regions.
Since memory is linear and contiguous, overflowing data overwrites critical components of memory, such as:
- Program instructions (e.g., return addresses in the stack).
- Data values (e.g., variables or function pointers).
Example of a Buffer Overflow
Here’s a simple example in C to illustrate the problem:
Key Problems in the Code:
- gets(): This function does not check the length of user input, so it writes beyond the bounds of the allocated buffer.
- strcpy(): This function also copies data into the buffer without size validation.
Scenario:
If a user inputs a string longer than 10 characters (e.g., 50 characters), the buffer will overflow. The excess data will spill into adjacent memory regions, potentially overwriting the program’s return address or other important values.
Example Input:
AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
Outcome:
The program’s execution could crash, or worse, attackers could inject malicious instructions that overwrite the return address to execute arbitrary code.
Types of Buffer Overflow Attacks
1. Stack-Based Buffer Overflow
In this type of attack:
- Buffers are allocated on the stack, which stores local variables and function call data.
- Overflowing the buffer can overwrite the return address, which determines the next instruction the CPU executes.
Goal: Overwrite the return address to point to malicious code injected by the attacker.
Example Attack:
An attacker sends input that overflows a buffer and overwrites the return address with the memory address of their payload (e.g., shellcode).
2. Heap-Based Buffer Overflow
In heap-based overflows:
- Buffers are allocated dynamically on the heap, the memory used for storing objects or dynamic data.
- Overflowing heap buffers can corrupt adjacent data or structures like function pointers.
Goal: Manipulate heap metadata or other structures to hijack program flow or corrupt data.
Exploitation of Buffer Overflow
Attackers exploit buffer overflows in multiple ways, depending on the application and operating system:
- Injecting Malicious Code
- Attackers overwrite the buffer with malicious instructions (e.g., shellcode).
- They manipulate the program’s return address to execute the malicious code.
- Return-Oriented Programming (ROP)
- Modern systems have protections like Non-Executable Stack (NX) that prevent code execution from memory regions like the stack.
- In response, attackers use ROP, chaining existing instructions in the program to perform malicious actions.
- Denial of Service (DoS)
- By overflowing buffers, attackers can cause programs to crash, leading to denial of service.
- Privilege Escalation
- Buffer overflow vulnerabilities in privileged processes (e.g., system services) can allow attackers to gain elevated permissions.
Why Buffer Overflows are Dangerous
1. Arbitrary Code Execution
Attackers can execute their own instructions on a target system.
2. System Compromise
Buffer overflow vulnerabilities can be used to gain complete control of a system.
3. Data Corruption
Overflowing buffers can corrupt program data, leading to unpredictable behavior.
4. Bypassing Security Controls
Exploiting buffer overflows can bypass authentication mechanisms or firewalls.
Real-World Examples of Buffer Overflow Attacks
1. Morris Worm (1988)
The Morris Worm exploited a buffer overflow in the finger
service on UNIX systems. It was one of the first major Internet worms and infected thousands of machines.
2. Microsoft Blaster Worm (2003)
This worm targeted a buffer overflow vulnerability in Windows DCOM RPC. It caused widespread denial of service and compromised millions of machines.
3. Heartbleed (2014)
Heartbleed exploited a buffer over-read vulnerability in OpenSSL. Though not a traditional overflow, it allowed attackers to access sensitive memory data.
Mitigation Techniques
1. Safe Programming Practices
- Use secure functions like
strncpy()
instead ofstrcpy()
to ensure size checks. - Avoid unsafe functions like
gets()
orscanf()
without length limits.
2. Bounds Checking
- Always validate the size of input before writing to buffers.
3. Compiler-Based Protections
Modern compilers provide protections such as:
- Stack Canaries: Special values placed before the return address to detect overflow.
- Address Space Layout Randomization (ASLR): Randomizes memory addresses to make exploitation harder.
4. Non-Executable Memory (NX)
Prevents execution of code from writable memory regions like the stack.
5. Static and Dynamic Analysis
Use tools like Valgrind, AddressSanitizer, and static code analysis to detect buffer overflows during development.
RAM Injection
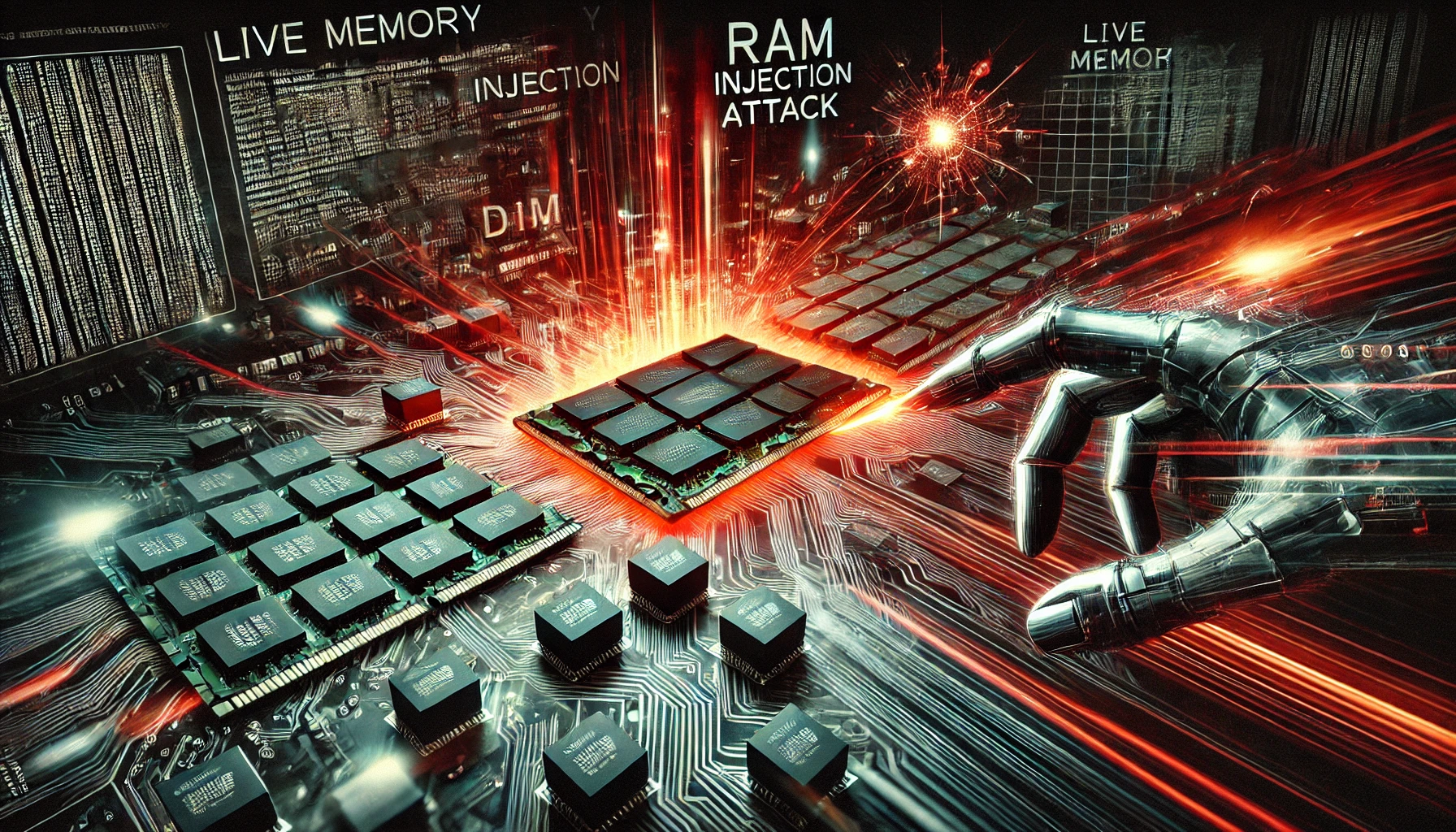
Definition
RAM Injection is a cyber attack where malicious code or data is directly injected into the system’s Random Access Memory (RAM). This method bypasses traditional disk-based malware detection since the payload operates entirely in live memory.
How RAM Injection Works
- In-Memory Execution: Attackers exploit processes to inject and execute malicious code directly in RAM.
- Diskless Attack: Since no files are saved to the disk, signature-based antivirus software often fails to detect the attack.
- Process Hijacking: The injected code can hijack legitimate processes, masking its malicious activity.
Techniques of RAM Injection
- DLL Injection
- Dynamic Link Libraries (DLLs) are injected into the memory of a legitimate process.
- The malicious DLL is executed in place of, or alongside, the original DLL.
- Tools like
Process Explorer
can sometimes reveal injected DLLs.
- Reflective DLL Injection
- The DLL is loaded directly into memory without using the standard Windows loader.
- This makes the injection harder to detect and avoids creating system logs.
- Process Hollowing
- Attackers replace the memory space of a legitimate process with malicious code.
- Example: Malware replaces the memory of
explorer.exe
with malicious payloads.
- Code Cave Injection
- Malicious code is placed into unused or “hidden” sections of a process’s memory, known as code caves.
- Shellcode Injection
- Small pieces of code (shellcode) are injected into memory and executed directly.
- Common in buffer overflow or ROP (Return-Oriented Programming) exploits.
Detection and Prevention
- Use Endpoint Detection and Response (EDR) tools to monitor suspicious in-memory activities.
- Enable DEP (Data Execution Prevention) to prevent code execution in unauthorized memory areas.
- Monitor processes and RAM usage for unexpected behavior using tools like Sysmon or Process Monitor.
RAM Spoofing
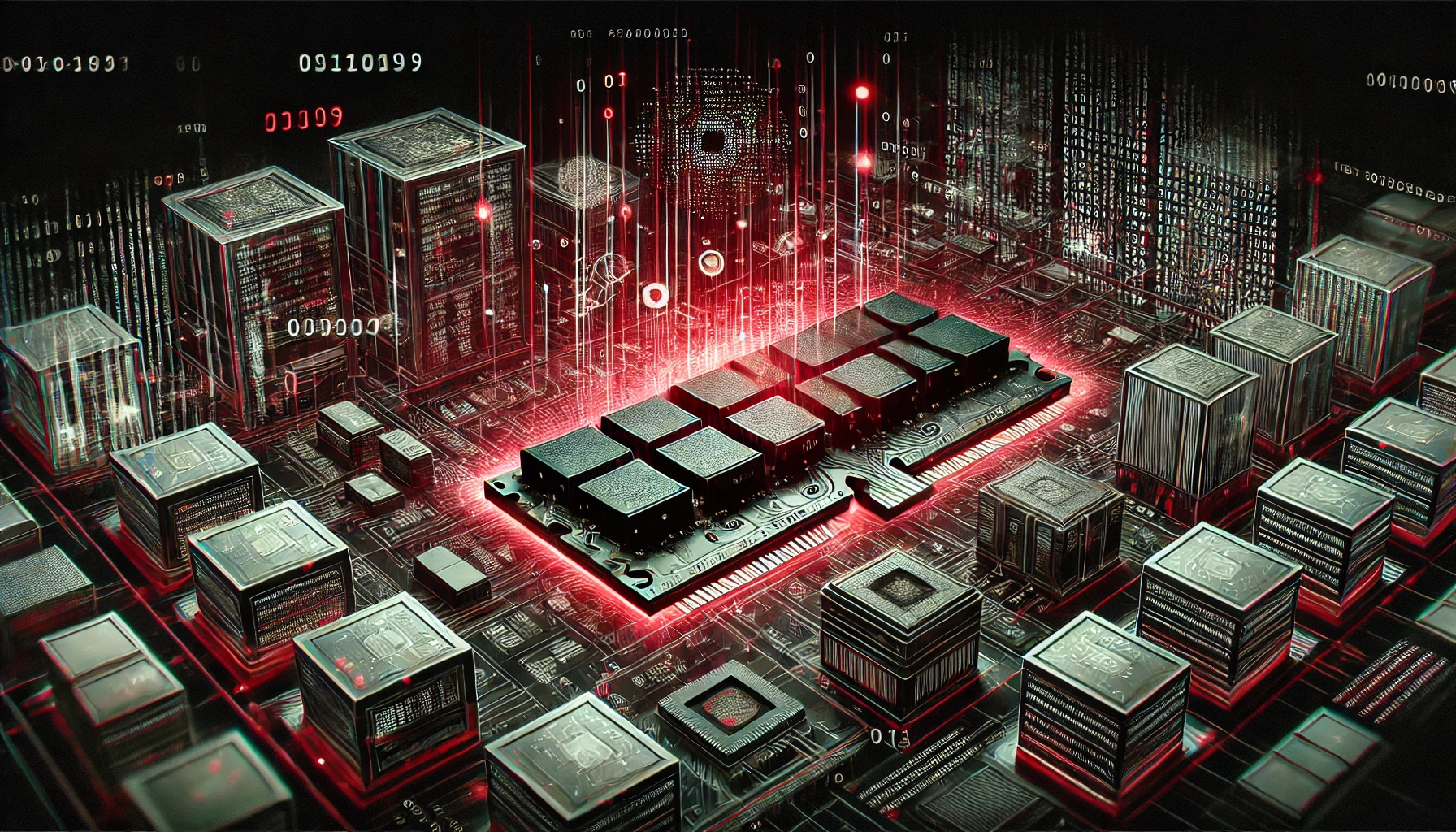
Definition
RAM Spoofing refers to tampering with or manipulating data stored in RAM to deceive the system or application. It often involves modifying runtime data to influence program behavior without touching static files or code on disk.
How RAM Spoofing Works
- Attackers access system RAM and modify key values, such as authentication tokens, game variables, or cryptographic keys.
- This is typically achieved through debugging tools, memory editors, or malware capable of interacting with RAM.
Examples of RAM Spoofing
- Cheating in Games
- Attackers use tools like Cheat Engine to modify values in RAM, such as health, ammunition, or game scores.
- Session Hijacking
- Spoofing authentication tokens or cookies stored in memory to impersonate legitimate users.
- Cryptographic Key Manipulation
- Extracting and altering cryptographic keys held in memory to bypass security protocols.
Detection and Prevention
- Use Trusted Execution Environments (TEEs), such as Intel SGX, to protect sensitive data in memory.
- Implement memory integrity checks and anti-debugging techniques.
- Deploy tools that continuously monitor for unusual changes in memory.
SQL Injection
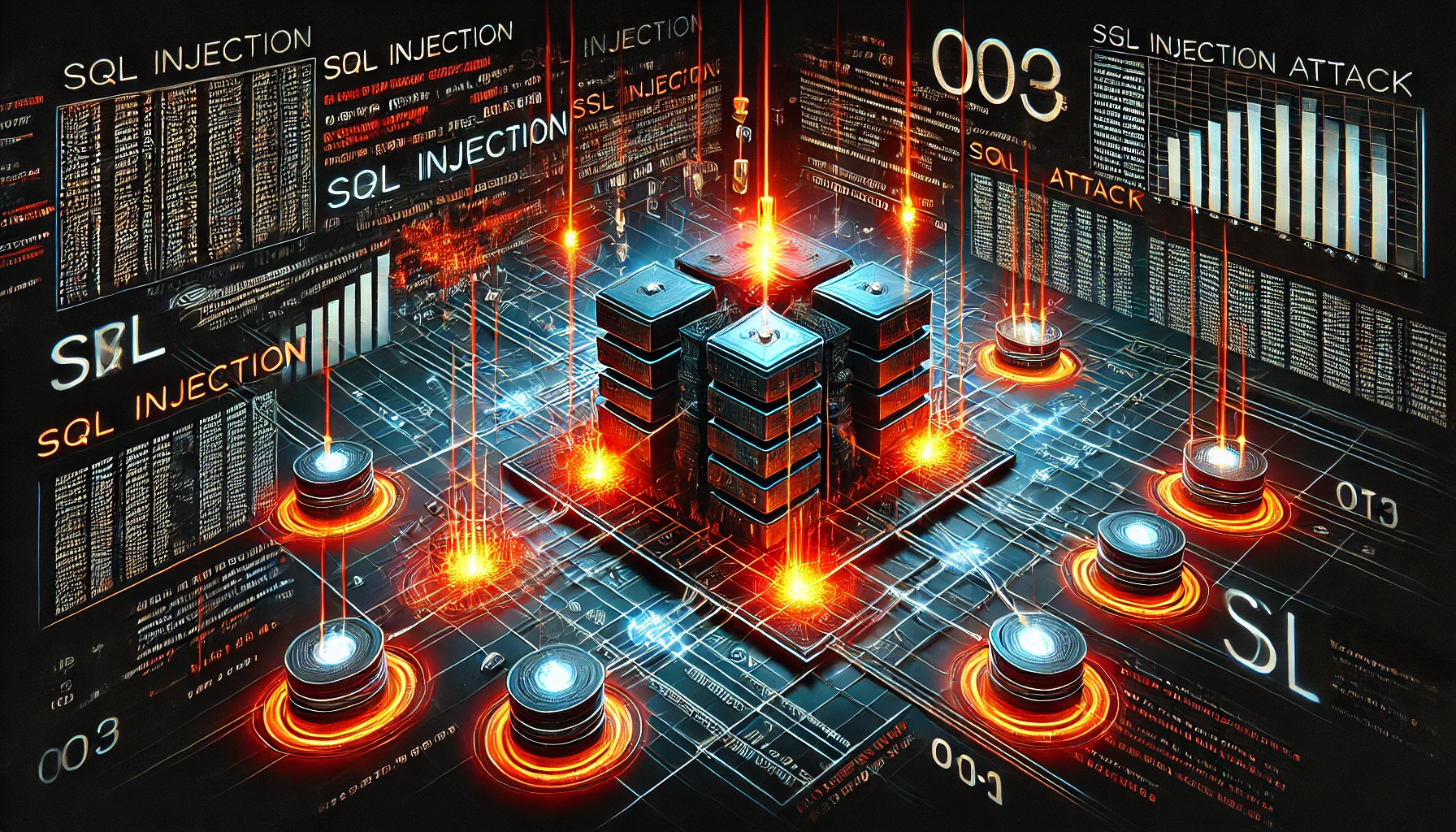
Definition
SQL Injection (SQLi) is a type of attack where malicious SQL code is injected into input fields to manipulate or exploit a database. It occurs when user inputs are improperly sanitized, allowing attackers to execute arbitrary SQL commands.
How SQL Injection Works
- User Input: The attacker inputs malicious SQL queries into web application fields (e.g., login forms, search boxes).
- Unsanitized Queries: The application improperly processes the input, allowing the SQL commands to run on the database.
- Database Manipulation: The attacker can retrieve, modify, or delete sensitive data, or gain administrative access.
Types of SQL Injection
- Classic SQL Injection
- Attackers inject SQL code to alter queries.
- Example:
Input:' OR 1=1; --
Query:
SELECT * FROM users WHERE username = '' OR 1=1; --
This returns all rows from the table.
- Blind SQL Injection
- Attackers cannot see query results but infer information based on application behavior.
- Example: If a condition is true, the page might load normally; otherwise, it might return an error.
- Error-Based SQL Injection
- Errors generated by invalid queries reveal database structure details.
- Example: Manipulating input to generate error messages containing table names.
- Union-Based SQL Injection
- Attackers use the
UNION
SQL operator to combine results from different queries. - Example:
SELECT column1, column2 FROM users UNION SELECT username, password FROM admin;
- Attackers use the
- Time-Based SQL Injection
- The attacker sends queries that cause database delays. Response times indicate whether conditions are true or false.
- Example:
SELECT IF(1=1, SLEEP(5), 'false');
Impacts of SQL Injection
- Data Breaches: Unauthorized access to sensitive data like usernames, passwords, or financial records.
- Data Corruption: Attackers can modify or delete database records.
- Privilege Escalation: Gaining administrative rights to the database.
- System Compromise: In some cases, attackers can execute system-level commands.
Prevention Techniques
- Parameterized Queries
- Use prepared statements with parameterized queries to separate SQL code from user input.
- Example in Python:
- Input Validation
- Validate and sanitize user inputs to ensure only expected data types are accepted.
- Use Stored Procedures
- Limit the execution of arbitrary queries by using pre-defined SQL procedures.
- Limit Database Privileges
- Restrict database user permissions to minimize the impact of potential attacks.
- Web Application Firewalls (WAF)
- Use WAFs to detect and block SQL injection attempts.
- Error Suppression
- Avoid displaying database error messages to users, as they can leak sensitive information.
Conclusion
Each of these attacks—RAM Injection, RAM Spoofing, SQL Injection, and Buffer Overflows—poses significant threats to system security, exploiting different aspects of system memory, runtime behavior, and database operations.
- RAM Injection focuses on live memory exploitation for stealthy code execution, bypassing traditional detection mechanisms.
- RAM Spoofing manipulates data stored in RAM to alter the behavior of processes at runtime.
- SQL Injection targets databases through malicious queries to compromise, manipulate, or delete sensitive data.
- Buffer Overflows exploit poor bounds-checking in code to execute arbitrary instructions, crash applications, or gain unauthorized access to systems.
Although some of these vulnerabilities have been known for years, they remain threats due to insecure coding practices, legacy systems, and evolving attack techniques. Understanding the mechanisms behind these attacks and implementing effective mitigation strategies—such as secure programming practices, modern defenses like stack canaries and ASLR, input validation, and endpoint monitoring—are essential to ensure systems and applications remain resilient against exploitation.